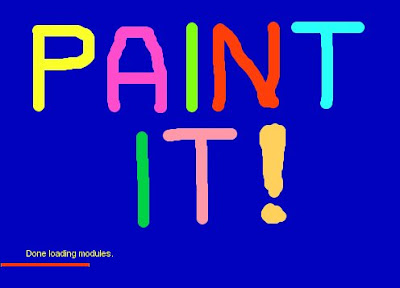
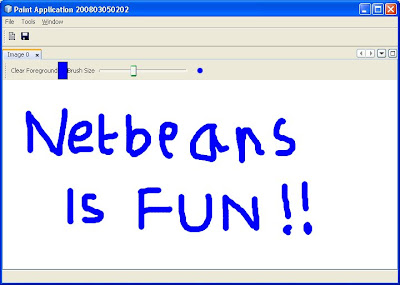
Like this,you have many applications. The others include the mobile application in mobility using game builder and many more applications in ruby,UML etc.
ABOUT THIS BLOG: My older posts deals with my interaction with netbeans. However, I am now going to branch off and delve into random technical problems I face in day-to-day work.
class Fun
{
public static void main(String[] args)
{
int count,i=0;
String words;
count=args.length;
System.out.println("Number of arguments= " +count);
while (i < count)
{
words=args[i];
i++;
System.out.println(i+ ":" + "Java is " +words+ "!");
}
}
}
This happens because the System class contains several class fields and methods and they cannot be instantiated. The facilities provided by the System class include standard input, standard output, and error output streams. By convention, this output stream is used to display information or messages that are intented to be attended by the user. "println" which is normally added at the terminal end belongs to the PrintStream. This class prints Java primitive values and object to a stream as text. Errors if present can be detected by calling the checkError() method. Additionally, this stream can be designated as "autoflush" when created so that any writes are automatically flushed to the underlying output sink when the current line is terminated. "println" just terminates the current line by inserting a line separator string(which does not mean \n).
class NewThread implements Runnable
{
String name;
Thread t;
NewThread(String threadname)
{
name=threadname;
t=new Thread(this,name);
System.out.println("New thread: " + t);
t.start();
}
public void run()
{
try
{
for(int i=15;i>0;i--)
{
System.out.println(name + ":" +i);
Thread.sleep(200);
}
} catch (InterruptedException e)
{
System.out.println(name + "interrupted");
}
System.out.println(name + "exiting");
}
}
class Accurate
{
public static void main(String args[])
{
NewThread ob1= new NewThread("One");
NewThread ob2= new NewThread("Two");
try{
Thread.sleep(1000);
ob1.t.suspend();
System.out.println("Suspending thread one");
Thread.sleep(1000);
ob1.t.resume();
System.out.println("Resuming thread one");
Thread.sleep(1000);
ob2.t.suspend();
System.out.println("Suspending thread two");
Thread.sleep(1000);
ob2.t.resume();
System.out.println("Resuming thread one");
} catch (InterruptedException e)
{
System.out.println("Main thread interrupted");
}
System.out.println("Main thread exiting");
}
}
To run this file,we click on Build->"Complile Accurate.java" (F9). After succesful compilation,we can run the program by clicking Run->Run file->Run "Accurate.java" (or Shift+F6). After this,we can observe the output which is
New thread: Thread[One,5,main]
New thread: Thread[Two,5,main]
One:15
Two:15
One:14
Two:14
One:13
Two:13
One:12
Two:12
One:11
Two:11
Suspending thread one
Two:10
Two:9
Two:8
Two:7
Two:6
Resuming thread one
One:10
Two:5
One:9
Two:4
One:8
Two:3
One:7
Two:2
One:6
Two:1
Suspending thread two
One:5
One:4
One:3
One:2
One:1
Resuming thread one
Twoexiting
Main thread exiting
Oneexiting
I suggest you to follow the steps carefully to format your code.
1) Select your entire program with the right click of a mouse ( the picture will be as shown above).
2) Right-click and click on the option which reads "format". The shortcut is Alt+Shift+F.
Simple,isn't it?? Just by following these two simple steps,one can arrive at a correct formatted code!
Right-click on the tab at facebook and click on "view API documentation". Why am I choosing facebook to explain my point? Well,today is the age of social networking and the chances that a person knows facebook is higher than the person knowing anything else!! So, your facebook application also makes method calls over the internet by sending HTTP requests to the facebook API server. This part of the option of netbeans is best learnt when the YOU choose to explore it! Like the tag line of netbeans 6.1 says..."the only IDE you need!!" Its open source! So guys,get started if you still haven't!!
vi) Now,we rename every display text component added on to the jFrame window. To do this, double click on every component and rename. The screenshot after doing so will be as shown below.
Just to make the photo more clearer,I have added an enlarged version of the photo. Hope it helps..!
Here it is important to observe that basic arithmetic operations can be performed on two operands wgich is indicated by 'First Number' and 'Second Number'. 'Unary field' is used when an operation is to be performed on a single number.
vii) After renaming is done,we need to add functionality to every component of the calculator. Let us start with the EXIT button. To add functionality,right click on the exit button and choose action and select action performed. The IDE will then open up the Source Code window. Now, scroll to where you implement the action you want the button to do when the button is pressed. Your source code window contains the following statement.
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt)
{ //TODO: Add your handling code here :
}
We add one line to exit and our final code looks as shown below. Similarly,one should follow the same procedure for adding functionality to different buttons and the codes for all buttons are as shown below.
I) EXIT Button
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt)II) SQUARE button:
{
System. exit(0);
}
private void jButton5ActionPerformed(java.awt.event.ActionEvent evt)
{
float num,result;
num=Float.parseFloat(jTextField3.getText());
result=num*num;
jTextField4.setText(String.valueOf(result));
}
III) MULTIPLY Button:
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt)
{
float x1,x2,result;
x1=Float.parseFloat(jTextField1.getText());
x2=Float.parseFloat(jTextField2.getText());
result = x1*x2;
jTextField4.setText(String.valueOf(result));
}
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt)
{
float x1,x2,result;
x1=Float.parseFloat(jTextField1.getText());
x2=Float.parseFloat(jTextField2.getText());
result = x1+x2;
jTextField4.setText(String.valueOf(result));
}
private void jButton4ActionPerformed(java.awt.event.ActionEvent evt)
{
float x1,x2,result;
x1=Float.parseFloat(jTextField1.getText());
x2=Float.parseFloat(jTextField2.getText());
result = x1/x2;
jTextField4.setText(String.valueOf(result));
}
private void jButton6ActionPerformed(java.awt.event.ActionEvent evt)
{
float num,result;
num=Float.parseFloat(jTextField3.getText());
result = 1/num;
jTextField4.setText(String.valueOf(result));
}
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt)
{
float x1,x2,result;
x1=Float.parseFloat(jTextField1.getText());
x2=Float.parseFloat(jTextField2.getText());
result = (x1-x2);
jTextField4.setText(String.valueOf(result));
}
private void jButton7ActionPerformed(java.awt.event.ActionEvent evt)
{
jTextField1.setText("");
jTextField2.setText("");
jTextField3.setText("");
jTextField4.setText(" ");
}
To compile build/compile the project, click on build->build main project.Then to run the main project, click on run-> run main project. When you do this, you can make calculations in this calculator! :)